Exception:
- Exceptions are the objects representing the logical errors that occur at run time and makes JVM enters into the state of "ambiguity".
- The objects which are automatically created by the JVM for representing these run time errors are known as Exceptions.
- An Error is a subclass of Throwable that indicates serious problems that a reasonable application should not try to catch. Most such errors are abnormal conditions.
- few of the subclasses of
Error
AnnotationFormatError
- Thrown when the annotation parser attempts to read an annotation from a class file and determines that the annotation is malformed.AssertionError
- Thrown to indicate that an assertion has failed.LinkageError
- Subclasses of LinkageError indicate that a class has some dependency on another class; however, the latter class has incompatibly changed after the compilation of the former class.VirtualMachineError
- Thrown to indicate that the Java Virtual Machine is broken or has run out of resources necessary for it to continue operating.- There are really three important subcategories of
Throwable
: Error
- Something severe enough has gone wrong the most applications should crash rather than try to handle the problem,- Unchecked Exception (aka
RuntimeException
) - Very often a programming error such as aNullPointerException
or an illegal argument. Applications can sometimes handle or recover from thisThrowable
category -- or at least catch it at the Thread'srun()
method, log the complaint, and continue running. - Checked Exception (aka Everything else) - Applications are expected
to be able to catch and meaningfully do something with the rest, such as
FileNotFoundException
andTimeoutException
.
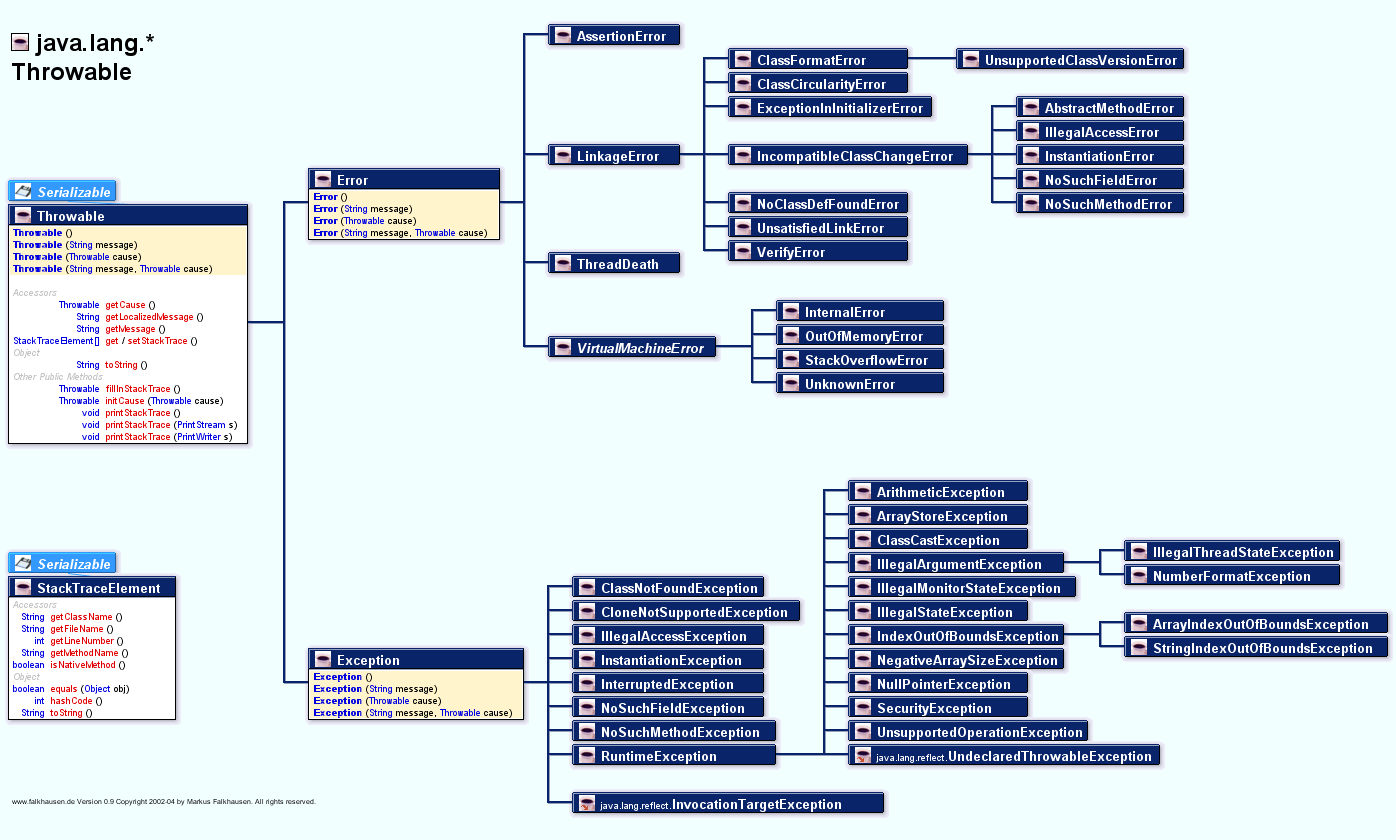
try block:
- The functionality of try keyword is to identify an exception object.
- And catch that exception object and transfer the control along with the identified exception object to the catch block by suspending the execution of the try block.
- All the statements which are proven to generate exceptions should be place in try block.
catch Block:
- The functionality of catch block is to receive the exception class object that has been send by the "try".
- And catch that exception class object and assigns that exception class object to the reference of the corresponding exception class defined in the catch block.
- And handle the exception that has been identified by "try".
- "try" block identifies an exception and catch block handles the identified exception.
finally block:
- finally blocks are the blocks which are going to get executed compulsorily irrespective of exceptions.
- finally blocks are optional blocks.
throw keyword:
- throw keyword used to throw user defined exceptions.(we can throw predefined exception too)
- If we are having our own validations in our code we can use this throw keyword.
- For Ex: BookNotFoundException, InvalidAgeException (user defined)
throws keyword:
- The functionality of throws keyword is only to explicitly to mention that the method is proven transfer un handled exceptions to the calling place.
- package com.instanceofjavaforus;
- public class ExcpetionDemo {
- public static void main(String agrs[]){
- try{
- //statements
- }catch(Exception e){
- System.out.println(e);
- }
- finally(){
- //compulsorily executable statements
- }
- }
- }
Unreachable Blocks:
- The block of statements to which the control would never reach under any case can be called as unreachable blocks.
- Unreachable blocks are not supported by java.
- Thus catch block mentioned with the reference of "Exception" class should and must be alwats last catch block. Because Exception is super class of all exceptions.
- package com.instanceofjavaforus;
- public class ExcpetionDemo {
- public static void main(String agrs[]){
- try{
- //statements
- }catch(Exception e){
- System.out.println(e);
- }
- catch(ArithmeticException e)//unreachable block.. not supported by java. leads to error
- System.out.println(e);
- }
- }
No comments