Posted by: InstanceOfJava
Posted date:
Dec 23, 2014
/
JDBC:
- The JDBC ( Java Database Connectivity) API defines interfaces and classes for writing database applications in Java by making database connections.
- Using JDBC you can connect to the database and execute any type of statements related relational database.
- JDBC is a Java API for executing SQL statements and supports basic SQL functionality.
JDBC Architecture:
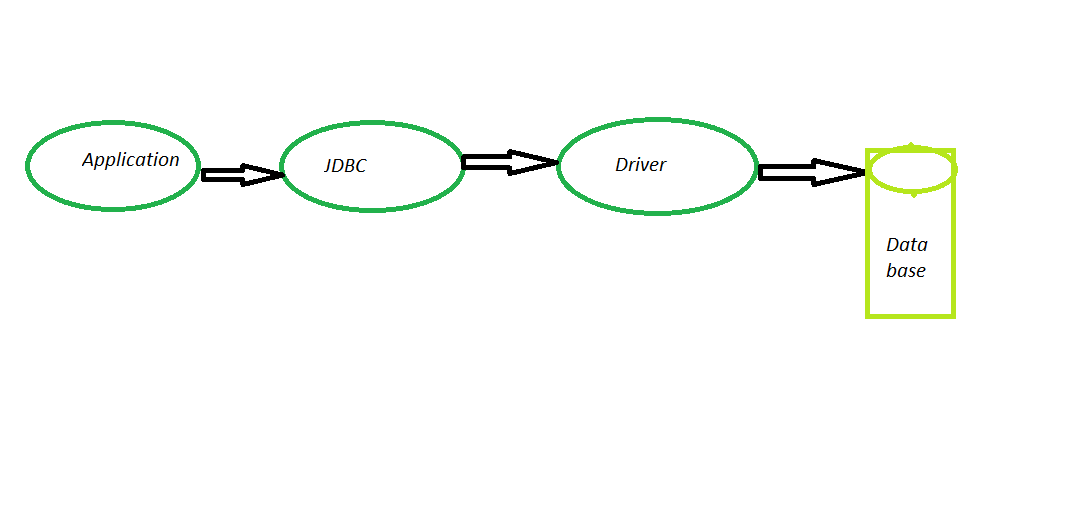 |
http://instanceofjavaforus.blogspot.in/
- Java application calls the JDBC API. JDBC loads a driver which connects to the database.
Connectivity Steps to JDBC:
5 Steps to connect java Application with the database using JDBC.They are:
- Register the driver class
- Creating Connection
- Creating Statement
- Execting Queries
- Closing Connection
Register the driver class:
- The forName() of class is used to register the driver class.Using this class is used load the class dynamically.
try {
Class.forName(com.mysql.jdbc.Driver”); //Or any other driver
}
catch(Exception x){
System.out.println( “Unable to load the driver class!” );
}
Creating the Connection:
- DriverManager is the backbone of JDBC architecture. DriverManager class manages the JDBC drivers that are installed on the system.
- Its getConnection() method of Driver Manager class is used to establish a connection to a database. It uses a username, password, and a jdbc url to establish a connection to
- the database and returns a connection object.
try{
Connection dbConnection=DriverManager.getConnection(url,”loginName”,”Password”)
}
catch( SQLException x ){
System.out.println( “Couldn’t get connection!” );
}
Creating Statement:
- Once a connection is established we can interact with the database. Connection interface defines methods for interacting with the database via the established connection. Statement
- A statement object is used to send and execute SQL statements to a database.
statement = dbConnection.createStatement();
Three kinds of Statements:
- Statement
- Prepared Statement
- Callable Statement
Statement:
- Execute simple sql queries without parameters.
Statement createStatement().
Prepared Statement:
- Execute precompiled sql queries with or without parameters.
PreparedStatement prepareStatement(String sql).
Callable Statement:
- Execute a call to a database stored procedure.
CallableStatement prepareCall(String sql)
Executing Queries:
- Statement interface defines methods that are used to interact with database and execution of SQL statements.
- The Statement class has three methods:
- executeQuery()
- executeUpdate()
- execute().
executeQuery():
- For a SELECT statement, the method to use is executeQuery .
executeUpdate():
- For statements that create or modify tables, the method to use is executeUpdate.
- statements and are executed with the method executeUpdate.
execute():
- execute() executes an SQL statement that is written as String object.
Connection Close():
- This method is used to close the connection.
con.close();
Syntax:
public void close() throws SQLException.
|
No comments